EOS Dev Guide 0x03
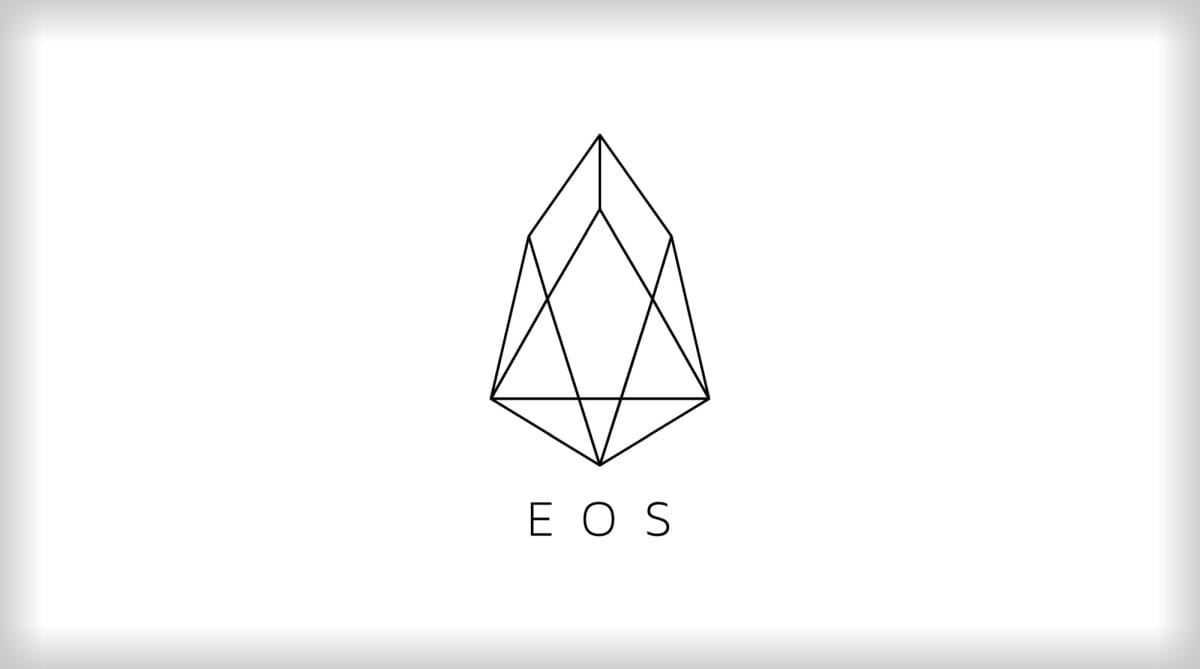
pre-requisite
This part assumes you've created at least one account.
If not, please check the previous post EOS Dev Guide 0x02.
Compile contract
contract needs to be first compiled to .wasm format as a bin file.
Then generate the .abi file to serve as a function interface index file.
create .cpp file
First, cd to any place and make a new folder to place the contract code.
$ mkdir -p ~/eos-dev/helloworld
$ cd ~/eos-dev/helloworld
$ vim helloworld.cpp
Then place the following code, save and quite(:wq)
#include <eosiolib/eosio.hpp>
#include <eosiolib/print.hpp>
using namespace eosio;
class helloworld : public eosio::contract {
public:
using contract::contract;
void hello(account_name user) {
print("Hello world! ", name{user});
}
};
EOSIO_ABI(helloworld, (hello))
compile to .wasm and .wast
$ eosiocpp -o helloworld.wast helloworld.cpp
This will generate .wast
and .wasm
at the same time..wast
file is the text version of .wasm
NOTE: if you encountered exceptions saying something like couldn't find file 'eosiolib/eosio.hpp', then try sudo eosiocpp -o ....
generate .abi file
$ eosiocpp -g helloworld.abi helloworld.cpp
alright, we've finished the preparation work.
Publish contract
In EOS, a contract needs to be attached to an account, functions would become actions
of that account.
For our test purpose, we are going to publish the helloworld contract on test.hello account and use it.
First, cd to helloworld/../
, which is the upper dir of our contract folder, in this example, it would be /eos-dev
$ cd /path/to/upper/dir/of/contract/folder
$ cleos set contract test.hello helloworld
Reading WAST/WASM from helloworld/helloworld.wasm...
Using already assembled WASM...
Publishing contract...
executed transaction: 6976f392d97728841a28ce7fb0e39734dd4ae58f9da5872cf9eeb3d2ddedd1f7 1808 bytes 569 us
# eosio <= eosio::setcode {"account":"test.hello","vmtype":0,"vmversion":0,"code":"0061736d01000000013b0c60027f7e006000017e600...
# eosio <= eosio::setabi {"account":"test.hello","abi":"0e656f73696f3a3a6162692f312e3000010568656c6c6f00010475736572046e616d6...
warning: transaction executed locally, but may not be confirmed by the network yet
Call contract function
$ cleos push action test.hello hello '["test.hello"]' -p test.hello
executed transaction: a0d226570d79330ea1e837e4c9da30080e92224cca83fbe564307bb7e873ac26 104 bytes 491 us
# test.hello <= test.hello::hello {"user":"test.hello"}
>> Hello world! test.hello
warning: transaction executed locally, but may not be confirmed by the network yet
NOTE: if you didn't get any output, check if you used --contracts-console
flag when starting nodeos. (ref)
And you'll see the same output in the nodeos
output.

$ cleos push action test.hello hello '["test.hello"]' -p test.hello
executed transaction: a0d226570d79330ea1e837e4c9da30080e92224cca83fbe564307bb7e873ac26 104 bytes 491 us
# test.hello <= test.hello::hello {"user":"test.hello"}
>> Hello world! test.hello
warning: transaction executed locally, but may not be confirmed by the network yet
End
Congrats! We've deployed and executed an EOS contract!
There are more contract examples under eos/build/contracts, the source code is under eos/contracts.
Alright, so much for this post, hope you enjoy.
Peace.