EOS Source Code Review - 0x00
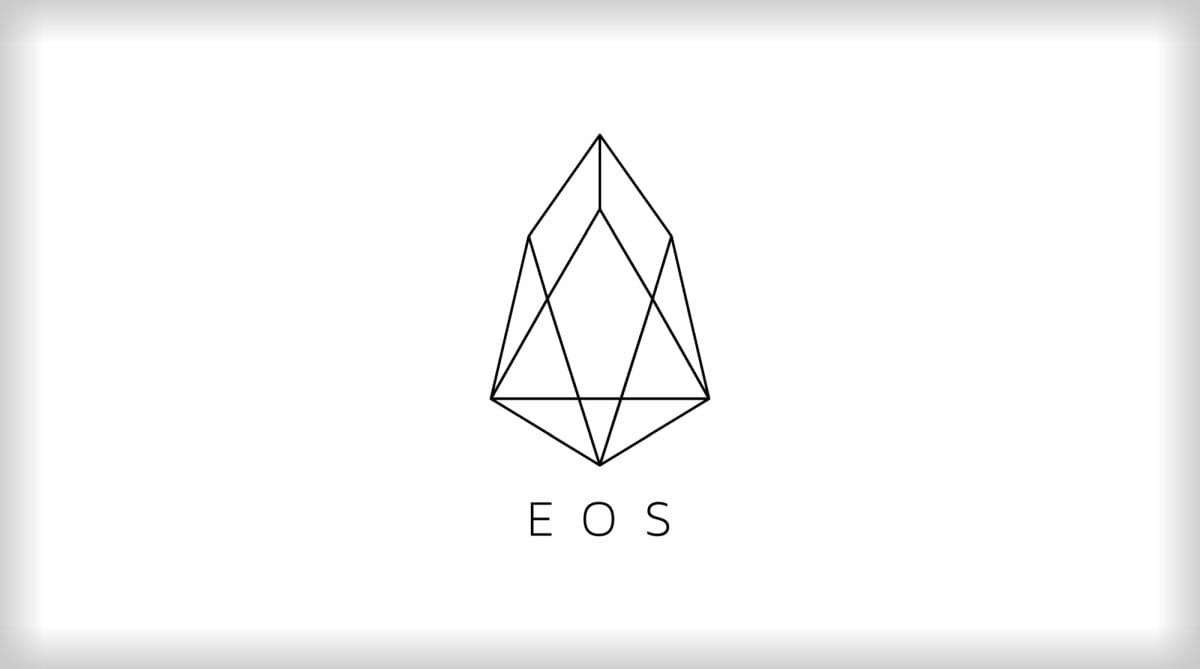
Before Start
I wrote this series of posts to remind myself the way I analysis EOS src code. My purpose was to learn security handling in C++.
I'll update posts as I analyzing it, so maybe it lacks of construction, forgive me on this if it does.
nodeos main.cpp
After I finished EOS deploying I know there are two important binaries, nodeos
and cleos
, nodeos contains the block producer logic, known as BP, while cleos acts as a client who could compile contract.cpp to contract.wasm and .wast, then deploy it to nodeos and nodeos could execute the .wasm and publish it to other BP as well as cleos nodes.
So the first thing I did was looking for the nodeos
logic. From the top to bottom, you know.
nodeos/main.cpp
First, it includes some files:
#include <appbase/application.hpp>
#include <eosio/chain_plugin/chain_plugin.hpp>
#include <eosio/http_plugin/http_plugin.hpp>
#include <eosio/history_plugin.hpp>
#include <eosio/net_plugin/net_plugin.hpp>
#include <eosio/producer_plugin/producer_plugin.hpp>
#include <eosio/utilities/common.hpp>
#include <fc/log/logger_config.hpp>
#include <fc/log/appender.hpp>
#include <fc/exception/exception.hpp>
#include <boost/dll/runtime_symbol_info.hpp>
#include <boost/exception/diagnostic_information.hpp>
#include "config.hpp"
files start with eosio
and fc
were from eos project, boost
were not.
(py3_env) ➜ eos git:(master) ✗ find . -name application.hpp
./libraries/appbase/include/appbase/application.hpp
(py3_env) ➜ eos git:(master) ✗ find . -name producer_plugin.hpp
./plugins/producer_plugin/include/eosio/producer_plugin/producer_plugin.hpp
(py3_env) ➜ eos git:(master) ✗ find . -name net_plugin.hpp
./plugins/net_plugin/include/eosio/net_plugin/net_plugin.hpp
(py3_env) ➜ eos git:(master) ✗ find . -name exception.hpp
./libraries/fc/include/fc/exception/exception.hpp
(py3_env) ➜ eos git:(master) ✗ find . -name common.hpp
...
./libraries/utilities/include/eosio/utilities/common.hpp
In main()
, it mainly operates app()
:
int main(int argc, char** argv)
{
try {
app().set_version(eosio::nodeos::config::version);
app().register_plugin<history_plugin>();
auto root = fc::app_path();
app().set_default_data_dir(root / "eosio/nodeos/data" );
app().set_default_config_dir(root / "eosio/nodeos/config" );
if(!app().initialize<chain_plugin, http_plugin, net_plugin, producer_plugin>(argc, argv))
return INITIALIZE_FAIL;
initialize_logging();
ilog("nodeos version ${ver}", ("ver", eosio::utilities::common::itoh(static_cast<uint32_t>(app().version()))));
ilog("eosio root is ${root}", ("root", root.string()));
app().startup();
app().exec();
} catch( ... ) {
...
}
}
app()
is from ./libraries/appbase/include/appbase/application.hpp
application.hpp:
namespace appbase {
namespace bpo = boost::program_options;
namespace bfs = boost::filesystem;
class application
{...
}
application& app();
...
}